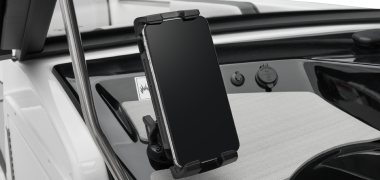
Wireless Charging
Wireless Charging
Conveniently charge your smartphone while on the water. Available on both the helm and the portside console.
You can choose up to four units.
The 22 foot platform comes with a deeper cockpit and greater freeboard, enabling a more spacious interior and larger bow area.
Discover The 22 Foot Lineup
The wide bow design includes beverage mounts and multiple bow seating configurations with backrest cushions for maximum comfort.
Portside Storage
Extra storage compartment for larger items
In Floor Storage
The deck and hull design maximizes space, comfort and versatility with bow filler cushions for multiple seating options.
Bow Seating Configurations
The deck and hull design maximizes space, comfort and versatility with bow filler cushions for multiple seating options.
Portside Storage
Extra storage compartment for larger items
In Floor Storage
The deck and hull design maximizes space, comfort and versatility with bow filler cushions for multiple seating options.
Bow Seating Configurations
The deck and hull design maximizes space, comfort and versatility with bow filler cushions for multiple seating options.
The top of the line 222SD features industry-first DRiVE® technology, E-Series® functionality and premium 12.3" Connext® touchscreen to put you in command like never before.
Yamaha's E-Series®
Features push-button start/stop, RPM engine sync, and single throttle lever pairing.
Connext® Touchscreen
Features 12.3-inch multi-color Connext touchscreen that is the hub for the entertainment functions, GPS mapping, and relevant boat system data when underway.
Wireless Charging
The 22 Foot Series features a smartphone holder with wireless charging at the helm and a wireless charging pad located portside.
Yamaha's E-Series®
Features push-button start/stop, RPM engine sync, and single throttle lever pairing.
Connext® Touchscreen
Features 12.3-inch multi-color Connext touchscreen that is the hub for the entertainment functions, GPS mapping, and relevant boat system data when underway.
Wireless Charging
The 22 Foot Series features a smartphone holder with wireless charging at the helm and a wireless charging pad located portside.
This new four-stroke, four-cylinder, 16-valve High Output Yamaha marine engine provides quicker and smoother acceleration and a higher top speed.
Yamaha's 22-foot boats give new meaning to the term "Fully Loaded".
Wireless Charging
Conveniently charge your smartphone while on the water. Available on both the helm and the portside console.
DRiVE Technology
Maneuver the boat forward and aft precisely in tight confines while keeping your hands on the steering wheel.
New Spacious Interior
Yamaha's "engineless" design frees up space in the cockpit and stern for seating, storage, and more.
Watersports Tower
The stylish, forward-sweeping, folding watersports tower is perfect for tow sports and tubing.
Yamaha's compact jet propulsion design sits low inside the hull, allowing for a spacious and versatile swim platform that is unrivaled in its class.
Swim Platform
Swim Up Seating
At anchor, two removable seats can be attached to the stern, providing comfortable in-water seating. All 22-foot runabouts come standard with mounts for optional swim-up seats (sold separately).
Swim Platform
Swim Up Seating
At anchor, two removable seats can be attached to the stern, providing comfortable in-water seating. All 22-foot runabouts come standard with mounts for optional swim-up seats (sold separately).